TicketSwift - Automated Ticket Purchaser
An automatic concert ticket purchaser for damai.cn
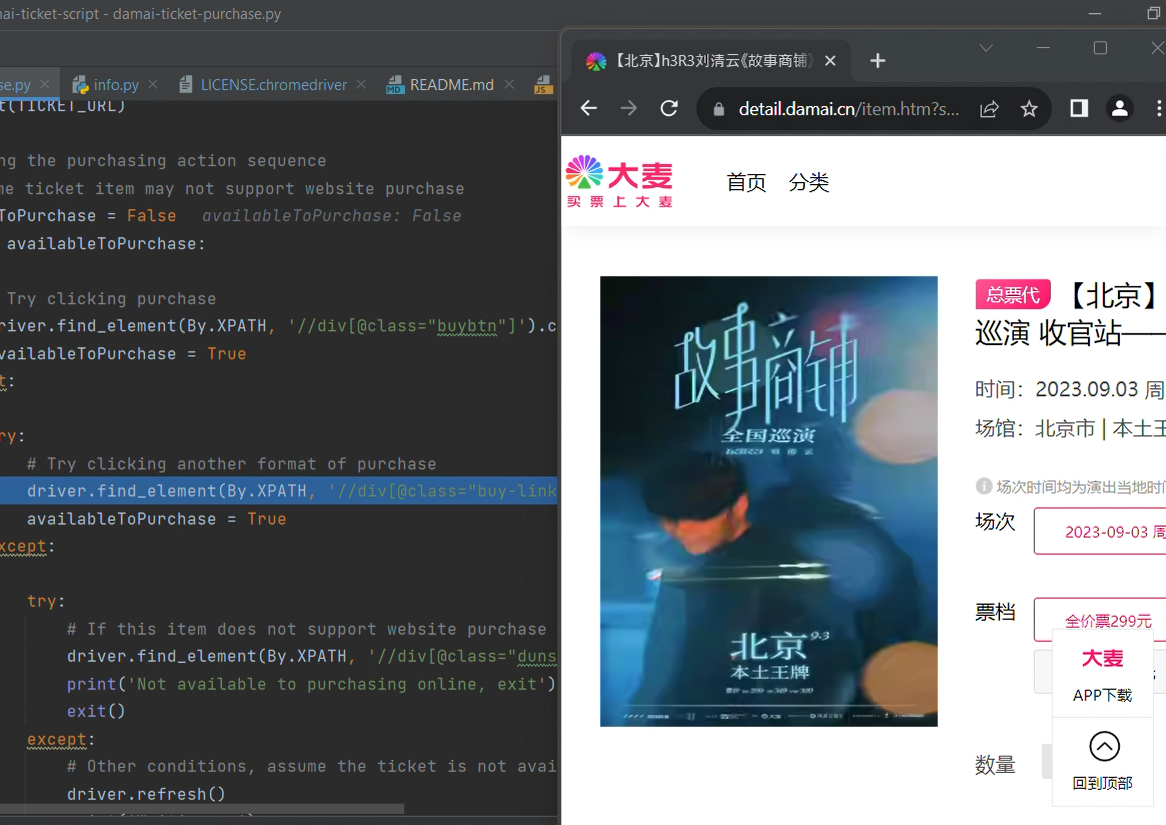
🌎 Final Product 👈
Backstory:
My cousin wanted to purchase a concert ticket on damai.cn; however, the tickets are usually sold within seconds when the ticket window opens. Thus, I am here to write a ticket purchaser script for him.
Investigation:
- I started by manually log in to damai.cn, used inspect to trace down the structure of the website.
- Knowing the correct path to the desired ticket purchasing location, I decides to use chrome webdriver travel through damai.cn.
- The website can be divided into three parts: login system, ticket window, and purchaser information; each comes up with a different challenge.
- Login system: Users need to go through an authentication system where they have to drag a slider over.
- Ticket window: A QR code may appear above purchase button, replacing the original pink purchase button.
- Purchaser information: users will be redirected to a purchase window where they have to manually enter their information.
- Login system: Users need to go through an authentication system where they have to drag a slider over.
Solution:
- Login system: use “click_and_hold” function to drag a slider over in a certain distance and time.
driver.switch_to.frame(0)
slider = driver.find_element(By.XPATH, '//*[@id="nc_1_n1z"]')
# Distance to slide over: 300-42=258
webdriver.ActionChains(driver).click_and_hold(on_element=slider).perform()
webdriver.ActionChains(driver).move_by_offset(xoffset=258, yoffset=0).perform()
webdriver.ActionChains(driver).pause(0.5).release().perform()
driver.switch_to.parent_frame()
- Ticket window: implement try-except block to go through different conditions.
try:
# Try clicking purchase
driver.find_element(By.XPATH, '//div[@class="buybtn"]').click()
except:
try:
# Try clicking purchase under QR code
driver.find_element(By.XPATH, '//div[@class="buy-link"]').click()
except:
try:
# If this item does not support website purchase
driver.find_element(By.XPATH, '//div[@class="dunsale"]')
exit()
except:
# Ticket is not available to purchase yet, refresh page
driver.refresh()
- Ticket window: use send_keys function to enter user info, like audience name.
driver.find_element(By.XPATH, PATH_TO_NAME_TEXTBOX).send_keys(NAME)
Conclusion:
I’m proud that I finished this project in about a week. Through this project, I’ve learned how to use selenium library and webdriver. Although there are still many improvements that can be made, having a small project like this is really enjoyable and fun. It lets me forget my course loads and allows me to submerge into the programming world 😎
Improvements:
- Due to region problem, if this code is executed outside China, a special phone-number identification is required. Users have to enter an identification code sent to their phones. This might be another great project to write a script entering user ID from phones.
- Different regions may cause different lagging time to the website, using “time.sleep()” might cause bugs, detecting website page availability using while loop would be better.